Creating robust and efficient code has always been the hallmark of a skilled developer. With the advent of AI-powered tools like GitHub Copilot, developers now have an intelligent pair programmer at their fingertips. In this article, we’ll delve into the intricacies of coding with GitHub Copilot, focusing on getting started, understanding its capabilities, and optimizing its usage for maximum efficiency.
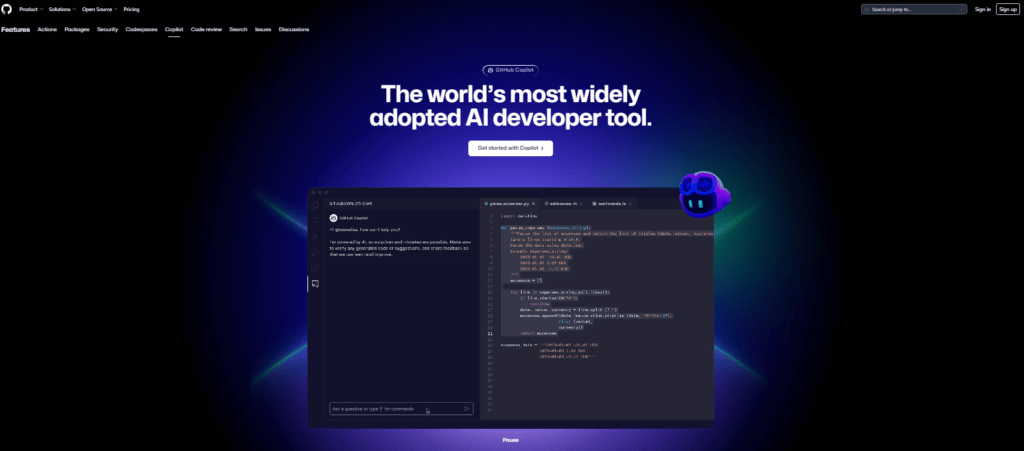
How GitHub Copilot Works
GitHub Copilot operates by sending the context of your code to GitHub. As you type, the tool analyzes the code and comments you’ve written and sends this context to GitHub Copilot. It utilizes the OpenAI language model behind the scenes, generating suggestions based on the provided context and sending them back to your IDE. You can accept, reject, modify, or use these suggestions as a foundation, allowing you to stay in the coding zone without frequently searching for syntax details.
Understanding Context in GitHub Copilot
To utilize GitHub Copilot effectively, it’s crucial to grasp what context it considers. First and foremost, the entire file you’re currently working on is sent as context, not just the portion above your cursor. This ensures a comprehensive understanding of your code.
Additionally, GitHub Copilot considers the tabs open in your IDE. It focuses on the files that are currently open, starting with the ones closest to the file you’re actively working on. This dynamic context allows Copilot to provide relevant suggestions based on your current coding session.
Tips for Maximizing GitHub Copilot’s Potential
1. Prompt Crafting: Setting the Stage
GitHub Copilot excels when you provide clear and specific prompts. Think of it as crafting a dialogue with your AI pair programmer. This process is termed “prompt crafting.” Let’s break down the components:
- Context: Clearly describe what you’re trying to achieve and how.
- Intent: Specify your actions, explaining the purpose and method.
- Clarity: Use straightforward language to ensure your intentions are easily understood.
- Specificity: Provide detailed information, avoiding ambiguity to get precise suggestions.
2. Best Practices for Prompt Crafting
a. Describe the Goal
Start your file with a few sentences describing the goal of your code. This provides context for Copilot, enhancing its understanding of your project.
b. Be Flexible
Understand that Copilot is probabilistic, not deterministic. If you don’t get the desired suggestion initially, consider rephrasing or modifying your prompt.
c. Provide Examples
Offering examples of what you’re looking for helps Copilot generate more accurate suggestions. If you’re working with data processing, include a few examples to guide the tool.
3. Name Things Properly: Follow Coding Best Practices
When crafting your code, GitHub Copilot pays attention to variable names. Ensure your variable names are descriptive, avoiding single-letter abbreviations. By following good coding practices, you make it easier for Copilot to generate relevant and readable code.
4. Open Relevant Files
If you’re not getting the suggestions you need, check if you have the relevant files open in your IDE. GitHub Copilot relies on the context provided by open tabs, so make sure you’re working with the appropriate files.
Putting it into Action: Building a Django Application
Now, let’s put these tips into action by building a Django application. Suppose we’re creating a conference website with speakers, tracks, and talks.
# Create a speaker model with name, email, and LinkedIn
class Speaker(models.Model):
name = models.CharField(max_length=255)
email = models.EmailField()
linkedin = models.URLField()
# Create a track model with name, description, and abbreviation
class Track(models.Model):
name = models.CharField(max_length=255)
description = models.TextField()
abbreviation = models.CharField(max_length=3, validators=[validate_uppercase])
# Create a talk model with title, description, speaker, track, and date
class Talk(models.Model):
title = models.CharField(max_length=255)
description = models.TextField()
speaker = models.ForeignKey(Speaker, on_delete=models.PROTECT)
track = models.ForeignKey(Track, on_delete=models.PROTECT)
date = models.DateField()
In this example, GitHub Copilot understands the context and generates relevant code snippets as we provide clear prompts and maintain good coding practices.
Conclusion
GitHub Copilot is a powerful ally in your coding journey. By understanding its inner workings and following best practices in prompt crafting, coding conventions, and file management, you can unleash its full potential. Treat it like a collaborative partner, and together, you’ll navigate the complexities of coding more efficiently.
In conclusion, embrace GitHub Copilot as a valuable tool in your developer toolkit, and let it enhance your coding experience. Happy coding!
One thought on “Unlocking the Power of GitHub Copilot: Tips and Tricks for Efficient Coding”